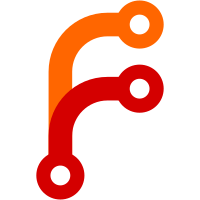
BREAKING CHANGE: Node v12.20+, v14.14+, or v16.0+ is required * fix!: remove esm package, use native Node ES modules * fix: fix some CJS imports
28 lines
986 B
JavaScript
28 lines
986 B
JavaScript
import { get } from '../../_utils/lodash-lite.js'
|
|
|
|
export function instanceMixins (Store) {
|
|
Store.prototype.getInstanceSetting = function (instanceName, settingName, defaultValue) {
|
|
const { instanceSettings } = this.get()
|
|
return get(instanceSettings, [instanceName, settingName], defaultValue)
|
|
}
|
|
|
|
Store.prototype.setInstanceSetting = function (instanceName, settingName, value) {
|
|
const { instanceSettings } = this.get()
|
|
if (!instanceSettings[instanceName]) {
|
|
instanceSettings[instanceName] = {}
|
|
}
|
|
instanceSettings[instanceName][settingName] = value
|
|
this.set({ instanceSettings })
|
|
}
|
|
|
|
Store.prototype.setInstanceData = function (instanceName, key, value) {
|
|
const instanceData = this.get()[key] || {}
|
|
instanceData[instanceName] = value
|
|
this.set({ [key]: instanceData })
|
|
}
|
|
|
|
Store.prototype.getInstanceData = function (instanceName, key) {
|
|
const instanceData = this.get()[key] || {}
|
|
return instanceData[instanceName]
|
|
}
|
|
}
|